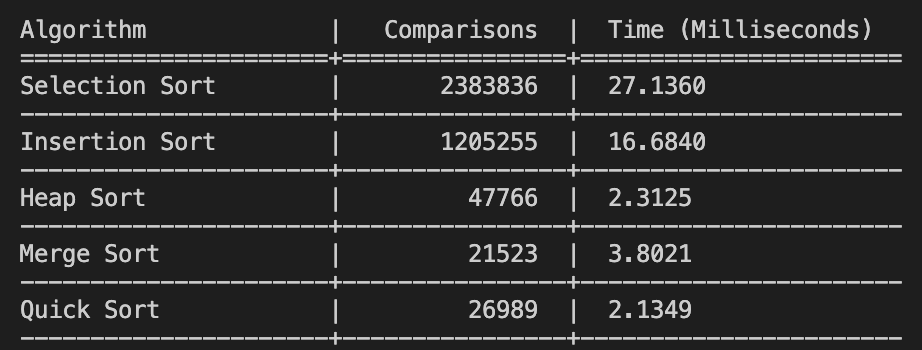
Comparing Sorting Algorithms
The purpose of this project is to understand how various sorting algorithms function as well as the number of comparisons and time required to complete each sort.
The sorting algorithms used are Selection Sort, Insertion Sort, Heap Sort, Merge Sort, and Quick Sort. The project was completed in Java.
See Full Code >